_workerToURL
converts a tiktag worker object into an usable Javascript-URL
Requires
import {_workerToURL} from "./tt/ttWorker.js";
Definition
function _workerToURL(ttWorkerObject)
Description
_workerToURL
is typically used to prepare a tiktag-worker class to be used in APIs like AudioWorklet
or a PaintWorklet
to be initialized with the addModule
member function. There are more sophisticated applications where _workerToURL
can be used turning your tiktag application into high speed multi-threading.
Parameters
ttWorkerObject
: A tiktag .worker Object
Return Value
[Url]
Remarks
-
Example: use worker to paint a rectangle on a canvas
main.js:
import {_newTag, _render} from "./tt/ttHTML.js";
import {_workerToURL} from "./tt/ttWorker.js";
import rectanglePaint from "./rectanglePaint.worker";
async function main()
{
var canvas = (<canvas style="border: 1px solid black" width="400" height="400"></canvas>);
_render(canvas);
await CSS.paintWorklet.addModule(_workerToURL (rectanglePaint));
canvas.style.setProperty("--rectangle-width", "100px");
canvas.style.background = "paint(rectangle-paint)";
}
main();
rectanglePaint.worker:
class rectanglePaint
{
static get inputProperties()
{
return ['--rectangle-width', '--rectangle-height'];
}
paint(ctx, size, props)
{
const width = parseFloat(props.get('--rectangle-width').toString()) || 50;
const height = parseFloat(props.get('--rectangle-height').toString()) || 50;
ctx.fillStyle = 'red';
ctx.fillRect(0, 0, width, height);
}
};
registerPaint('rectangle-paint', rectanglePaint);
Output:
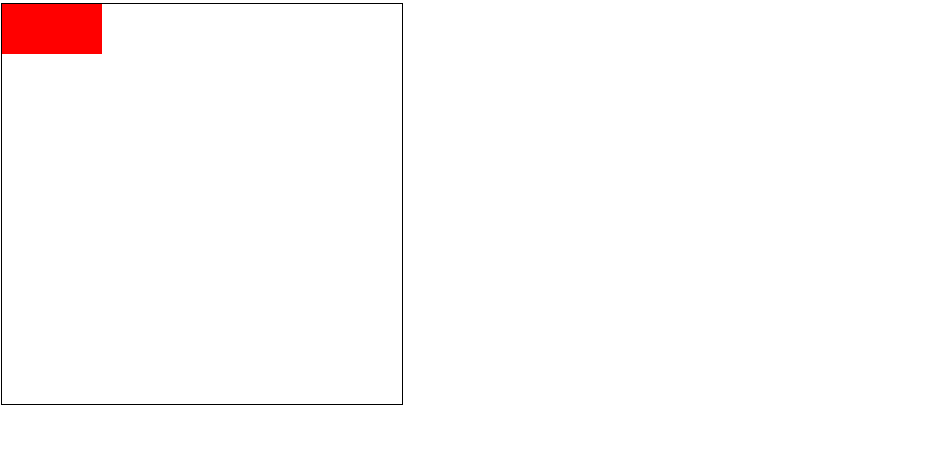