malloc
allocate a buffer and return base-pointer
Requires
#include "tiktag.h"
Definition
void* malloc(unsigned int size);
Description
The malloc()
function is used to dynamically allocate memory at runtime. It stands for "memory allocation" and is defined in the
The malloc() function takes a single argument, which is the number of bytes to allocate. It returns a pointer to the first byte of the allocated memory block
Parameters
unsigned int size
: size of the desired buffer in bytes
Return Value
void*
Remarks
The WebAssembly runtime provides a memory management system that allows programs to allocate memory dynamically, while ensuring that memory access is safe and secure.
In the WebAssembly memory model, memory is represented as a linear array of bytes that can be accessed using load and store instructions. The size of the memory array is fixed at the time the module is instantiated and cannot be changed at runtime. However, the module can dynamically allocate memory within this array.
The WebAssembly runtime provides a sandboxed execution environment that isolates the module from the rest of the system. This sandboxing approach ensures that WebAssembly modules cannot access or modify memory outside of their own linear memory array, which helps prevent security vulnerabilities such as buffer overflow attacks.
In addition to sandboxing, the WebAssembly runtime also provides runtime validation of WebAssembly modules to ensure that they are well-formed and safe to execute. This validation includes checking the types of function parameters and return values, verifying that memory accesses are within bounds, and ensuring that the module does not use any unsafe instructions or undefined behavior.
Overall, the WebAssembly runtime provides a secure and efficient environment for running low-level code in a variety of contexts, including web browsers, server-side applications, and embedded systems. The memory management system and sandboxing approach ensure that WebAssembly modules can allocate and manage memory dynamically without compromising the security and integrity of the system.
Example: generate and display random numbers in C++ using malloc - Buffer
main.js:
import {_newTag, _render, _setParentObject} from "./tt/ttHTML.js";
import {_wasmInitA} from "./tt/ttWASM.js";
import wasm from "./web.wasm";
const nNumbers = 100;
function jsGetSeed()
{
return (Date.now());
}
function jsRenderNumber(number)
{
_render(<li>{number}</li>);
}
async function main()
{
var wasmInstance = await _wasmInitA (wasm, {jsGetSeed: jsGetSeed, jsRenderNumber: jsRenderNumber}, null);
_render(<h1>Random Number Demonstration for malloc</h1>);
var list = <ul></ul>;
_render(list);
_setParentObject(list);
wasmInstance.exports.genRandomNumbers(nNumbers);
wasmInstance.exports.drawRandomNumbers(nNumbers);
}
main();
myCppExample.cpp:
#include "tiktag.h"
_import unsigned int jsGetSeed();
_import void jsRenderNumber(int number);
int* _numberBuffer;
unsigned int _seed;
unsigned int _lastNumber;
int getRandom()
{
if ((_lastNumber&0x02040010) != 0)
_seed -= 139812284;
unsigned int vec = _seed * (_lastNumber^0xa525a35c);
if ((vec&1) != 0)
vec = (vec>>1)|0x80000000;
else
vec >>=1;
vec = vec * 1103515245 + _seed;
_lastNumber = vec;
return vec;
}
_export void genRandomNumbers(unsigned int amount)
{
int i;
_seed = jsGetSeed();
_numberBuffer = (int*) malloc (amount*sizeof(int));
for (i=0;i<amount;i++)
_numberBuffer[i] = getRandom();
}
_export void drawRandomNumbers(unsigned int amount)
{
int i;
for (i=0;i<amount;i++)
jsRenderNumber(_numberBuffer[i]);
}
Output:
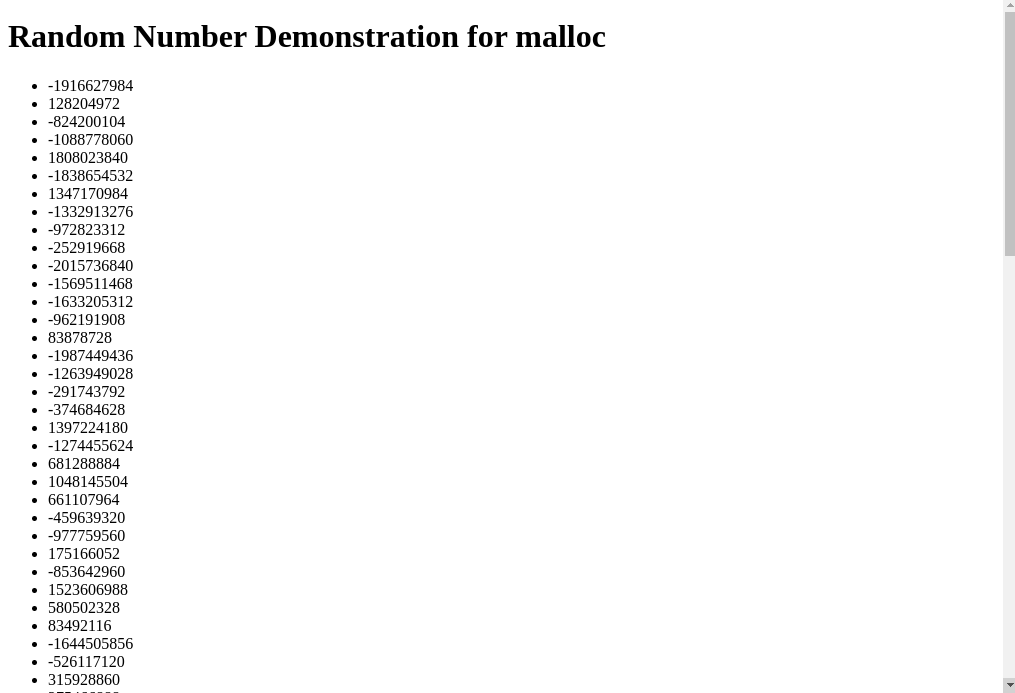